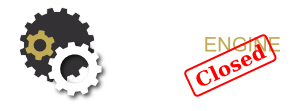
Wednesday, August 25. 2010
Xcode and 'Fix and Continue'
A quick pro tip:
Coming from the Java world and the eclipse IDE, I fell in love with the 'Drop to Frame' feature and the ability to change code on the fly while debugging. In Xcode, you can get half way there with the 'Fix' command (under the Run menu, enabled only while paused while debugging). The frustrating thing is that in the most recent version of Xcode (v3.2.3) this command is constantly disabled.
If you read the Apple's "Xcode Debugging Guide" (http://developer.apple.com/mac/library/documentation/DeveloperTools/Conceptual/XcodeDebugging/230-Modifying_Running_Code/modifying_running_code.html) and follow its guidelines about how to set up your environment to enable the menu item, it'll most likely stay disabled. The thing that the guide doesn't mention, and therefore is probably a bug and one that hopefully gets patched in Xcode 4, is that your build architecture must be 32-bit only. Since the default architecture is 32/64 bit universal, the fix command is unavailable by default until you change it.
Wednesday, August 18. 2010
LAN Aftermath Part 2
Friday, August 13. 2010
Passing pipes to subprocesses in Python in Windows
Passing pipes around in Windows is a bit more complicated than it is in Unix-like operating systems. This post is a bit of information about how file descriptor inheritance works in Windows and a quick example of how to do it.
The first difficulty to overcome is that file descriptors are not inherited by subprocesses in Windows as they are in Linux. However, OS file handles can be inheritable, and it is possible to retrieve the OS file handle associated with a C file descriptor using the _get_osfhandle function. It is also possible to convert the OS file handle back to a C file descriptor in the child process using _open_osfhandle. However, the OS file handle is not inheritable (see Python Bug 4708 - although on a side note, I don't think they should be default-inheritable since there is no preexec_fn in which to close them and prevent deadlock situations where a child holds the write end of a pipe open, but that is another matter), so to get an inheritable OS file handle it must be duplicated using DuplicateHandle.
The operating system-specific functions described above can be accessed through the _subprocess and msvcrt modules. Their use can be seen in the source for the subprocess module, if desired. However, note that the interface in _subprocess is not stable and should not be depended on, but since there is no alternative (that I am aware of - short of writing another module in C) it is the best solution. Now, an example:
parent.py:
import os
import subprocess
import sys
if sys.platform == "win32":
import msvcrt
import _subprocess
else:
import fcntl
# Create pipe for communication
pipeout, pipein = os.pipe()
# Prepare to pass to child process
if sys.platform == "win32":
curproc = _subprocess.GetCurrentProcess()
pipeouth = msvcrt.get_osfhandle(pipeout)
pipeoutih = _subprocess.DuplicateHandle(curproc, pipeouth, curproc, 0, 1,
_subprocess.DUPLICATE_SAME_ACCESS)
pipearg = str(int(pipeoutih))
else:
pipearg = str(pipeout)
# Must close pipe input if child will block waiting for end
# Can also be closed in a preexec_fn passed to subprocess.Popen
fcntl.fcntl(pipein, fcntl.F_SETFD, fcntl.FD_CLOEXEC)
# Start child with argument indicating which FD/FH to read from
subproc = subprocess.Popen(['python', 'child.py', pipearg], close_fds=False)
# Close read end of pipe in parent
os.close(pipeout)
if sys.platform == "win32":
pipeoutih.Close()
# Write to child (could be done with os.write, without os.fdopen)
pipefh = os.fdopen(pipein, 'w')
pipefh.write("Hello from parent.")
pipefh.close()
# Wait for the child to finish
subproc.wait()
child.py:
import os
import sys
if sys.platform == "win32":
import msvcrt
# Get file descriptor from argument
pipearg = int(sys.argv[1])
if sys.platform == "win32":
pipeoutfd = msvcrt.open_osfhandle(pipearg)
else:
pipeoutfd = pipearg
# Read from pipe
# Note: Could be done with os.read/os.close directly, instead of os.fdopen
pipeout = os.fdopen(pipeoutfd, 'r')
print pipeout.read()
pipeout.close()
Note: For this example to work, python must be on on the executable search path (i.e. in the %PATH% environment variable). If it is not, change the subprocess invocation to include the full path to python.exe. Note also that there is some complication with OS file handle leakage. When a _subprocess_handle object is garbage collected the handle that it holds is closed, unless it has been attached. Therefore, if the subprocess will outlive the scope in which the handle variable is defined, it must be detached (by calling its Detach method) to prevent closing during garbage collection. But this solution has its own problems as there is no way (that I am aware of) to close the handle from the child process, which means a file handle will be leaked.
Above caveats aside, this method does work for passing pipes between parent and child processes on Windows. I hope you find it useful.
Monday, August 9. 2010
BAD_POOL_HEADER in VirtualBox
Thursday, August 5. 2010
Prevent Web Pages from Stealing Focus
I have had enough of web pages arbitrarily changing the cursor focus. Enough of typing half of a URL into the URL bar and half into the text box that stole focus. Enough of typing half of a password into a password box and half into the text box that stole focus. Enough! If you have had enough, check out the NoFocus Greasemonkey script. This script prevents pages from changing focus during page load and, optionally, for the entire life of the page.
This is not a new problem. It has been discussed before on superuser and elsewhere. There are also alternative solutions, such as using Firefox's Configurable Security Policies to disallow focus, or using another Greasemonkey script such as NoFocusForYou and Disable Focus on Window Load. However, NoFocus has several advantages:
- No exceptions are thrown, as with CSPs. Most page authors are not expecting focus to throw exceptions (rightly so), and when this happens it will often completely break the pages by preventing the remainder of the script from running.
- Disables focus immediately (well, in the DOMContentLoaded event, when Greasemonkey runs its scripts). Other scripts only disable focus changing once the load event has fired, which doesn't address focus changes before this event occurrs.
- Disables focus changes for all elements currently on the page and all dynamically created elements. Rather than disabling focus for the elements on the page during load or DOMContentLoaded, this script disables focus for all elements current and future.
- Provides warnings when focus change is prevented. Inevitably, when debugging a page sometime in the future, we will forget that focus changing is prevented (if only briefly) and will fail to understand why a page is not working as expected. NoFocus counteracts this problem by using the Greasemonkey logging to write a warning message into the error log that focus change has been prevented. Making it easier to determine why focus is not performing as expected.
I hope you enjoy the script and enjoy not losing focus!
Sunday, August 1. 2010
State of the company August 1st
The blog has recently started to look like it was written by programming robots. To show that we are actually real life people with feelings and emotions and organs I figured I would write a little about what we have been up to.
Wednesday was the Emerging Technologies Symposium hosted by the Chamber of Commerce. We all went and got to talk with some cool folks. I got us a booth right across from Microsoft thinking Kevin could wage an all-out war in order to release some of the pent up frustration he has had building since he started working with the Microsoft Sync framework. Unfortunately Microsoft sent a non-Microsoft lackey probably realizing full-well that we were planning on ambushing them. Next time Microsoft! Otherwise I thought it was an excellent way to advertise and spend the day. Especially considering the booth only cost $150 and we all got lunch+$25 gift card from HP.
What We Are Doing Right Now
Peter is finishing up work on the heat mapping software he has been working on for NWB Sensors. If you need the best heat camera+software solution that money can buy contact one of the guys at NWB! Kevin has been working hard on the big office management suite he is making for HCS as well as on a super secret project demo. As I mentioned above some of the office management suite functionality was being done using Microsoft Sync but I'm pretty sure Kevin gave up on that and moved back to Git which is great because we now have office t-shirts that say "Git Forked" and this is just one more reason to wear them. I have been working on a PHP script to download MLS files from a repository and add property listings to a Joomanager database as well as create search functionality for said database. Unfortunately because Joomanager is set up to allow you enter any number of fields you want dealing with the database is a gigantic pain. Fortunately it is a kind of fun challenge and I'm almost done!
What We Will Do In The Future (Probably)
This Friday the 6th is our second LAN party. We will be holding it at the Bozeman public library from 3:00 p.m.-Midnight'ish and the theme is StarCraft II but everyone and all games are welcome. Our official LAN website is: http://www.digitalenginesoftware.com/lan/ . Further out I will be helping to teach classes in rural Montana towns on how to setup and market your small business website. This program is being put on by the Tech Ranch and you can find out more at the Orbit Montana website. Peter will be working on our speculative project which is just getting to the exciting parts and Kevin will be planning his revenge. I'll update more next month!