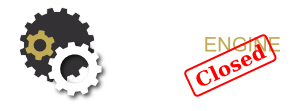
Wednesday, October 21. 2009
JavaScript image rotator
In an effort to make our page a little more personal and colorful I added an image rotator to our index page with pictures that I had taken around our office. There are about five million rotator scripts on the internet but I hadn't posted to the blog in a while so here is mine!
<html>
<body onLoad = "setImg();"><!-- Calls the setImg() function after the page has loaded -->
<span id="images"></span><!-- Image html will appear between these spans when the onLoad event is triggered -->
<script type="text/javascript">
//This is the array where you store the location of the pictures you would like to rotate through.
var imageArr = new Array("gfx/artofprogramming_small.jpg","gfx/thinkpad_small.jpg","gfx/theoffice_small.jpg","gfx/whiteboard_small.jpg","gfx/park_small.jpg","gfx/apple_small.jpg");
var numImages = imageArr.length;
function setImg(){
var randomNumber=Math.floor(Math.random()*numImages);//Selects a random number between 0 and the number of images in the array minus 1
document.getElementById("images").innerHTML = "<img src ='"+imageArr[randomNumber]+"' alt='rotating images from the office' />"; //Adds img html to your page between the two elements with id="images" }
</script>
</body>
</html>
Friday, October 16. 2009
HOWTO Modify Certificate Templates in SBS 2003
When using the Certificate Template MMC Snap-In to modify certificate templates in Windows Server 2003, any modifyable template is saved in the Version 2 certificate template format, which can only be used by Windows Server 2003 Enterprise Edition. This creates a significant annoyance when attempting to use certificate templates on a non-Enterprise Edition server (such as Small Business Server). However, when copying a Version 1 template (and making only minor modifications), there is no reason that the template can't be used as a Version 1 template, if you make some modifications by-hand.
Case in point: Setting up offline L2TP/IPSec Certificate Templates. If you have been following KB 555281, you are likely stuck at How to issue the custom L2TP/IPSec (Offline request) template. Here's the trick:
- Setup ADSI Edit, or any other LDAP editor
- Open adsiedit.msc (or your LDAP editor) and browse to CN=Configuration,CN=Services,CN=Public Key Services,CN=Certificate Templates
- Open the Properties for the template that you would like to use
- Change msPKI-Template-Schema-Version and msPKI-Template-Minor-Revision from 2 to 1 (not sure if msPKI-Template-Minor-Revision is really required...)
- Refresh the Certification Authority MMC Snap-In if it is open
That's it. If the template version is set to 1, you can issue the template in any edition of Server 2003.
Note: If you make substantial changes to the properties of the template this trick may not work. The differences in how the template versions are processed can be significant, but this process is likely to work for most simple changes to an existing Version 1 tepmlate.
A Warning to SonicWall Users about IP Fragmentation
Recently I discovered and corrected an obscure problem on a client's system relating to SMTP mail not being received from a single remote domain. The ultimate cause turned out to be the cause for an earlier (only partially solved) problem relating to POST data getting lost for the server hosting their website, and it is all the result of the default configuration on their SonicWall firewall.
By default, SonicWall will block/discard fragmented IP packets. This can lead to very difficult to diagnose problems as large packets (packets larger than the MTU of any link between the source and destination) will mysteriously fail to arrive. To solve the problem, follow the instructions to re-enable fragmented packets.
Note: The reason that fragmented packets are disabled by default is reasonable (at least for simple IP implementations). An IP implementation must keep track of fragments received but not yet reassembled so that when other fragments of the packet arrive (possibly much later and out of order) the original packet can be reassembled. Attackers can use this fact to contribute to a DoS attack by sending many packet fragments which do not contribute to complete packets. This will force the victim system to hold the fragments in memory and exhaust system resources. However, I would expect all practical IP implementations have a limited cache size for fragments to mitigate this scenario...
In summary, I find this default configuration completely unacceptable. A "break the Internet" default policy is ridiculous. I'm surprised that this hasn't bitten more people and wasted more time (or that the affected people haven't complained more loudly about their wasted time). Perhaps it is just Montana that is still using carrier pigeons and other forms of transport with small MTUs...
Monday, October 5. 2009
Broken Date.format in Microsoft ASP.NET 2.0 AJAX
The most recent version of the Microsoft ASP.NET 2.0 AJAX Extensions 1.0 (1.0.61025 according to the support info. in Add/Remove programs) has a bug that just bit me. This library adds a format function to the Date object which provides support for Date and Time Format Strings comparable to the .NET implementation. This is a very handy feature, given JavaScript's lack of a built-in date formatting function. But, if you are going to use it, be careful about the following bug: The time zone offset formats have incorrect sign. For example, if you are in the Pacific Time Zone at UTC-8, Date.format will return formats with UTC+8 (which matches the sign of JavaScript's Date.getTimezoneOffset method).
I created a workaround for the websites that I maintain which does not reimplement Date.format (and likely introduce new bugs) nor require any changes to existing code (with one exception - noted below). This code is a horrible hack and I am almost too embarrassed to post it... but not quite. Here it is:
(function() {
var dfmt;
function fixedDateFormat(format) {
var gto = Date.prototype.getTimezoneOffset;
try {
Date.prototype.getTimezoneOffset = function() { return -gto.call(this); }
return dfmt.call(this, format);
} finally {
Date.prototype.getTimezoneOffset = gto;
}
}
var fixfails = 0;
function fixIt() {
dfmt = Date.prototype.format;
if (typeof(dfmt) != "function") {
// If the library has not been loaded yet, defer
if (++fixfails < 3)
setTimeout(fixIt, 100);
return;
}
if (new Date().getTimezoneOffset() > 0 == new Date().format("zz") > 0)
Date.prototype.format = fixedDateFormat;
}
fixIt();
})();
Just include this code somewhere on the page and it will wrap the Date.format function and negate Date.getTimezoneOffset so that the formatted offset is correct. The significant limitation of the code is that if it is included before Date.format is defined, it will wait for the code to load and try again later. The problem with this approach is that code which is run after Date.format is defined before the fix is installed will still trigger the bug and it will be hard to figure out why that code is not working. Therefore, if possible, include this code immediately after the Microsoft AJAX extensions JavaScript is loaded on the page. Alternatively, use your own workaround or avoid using any of the "z" specifiers in your custom format strings.